Variables in JavaScript: var, let and const
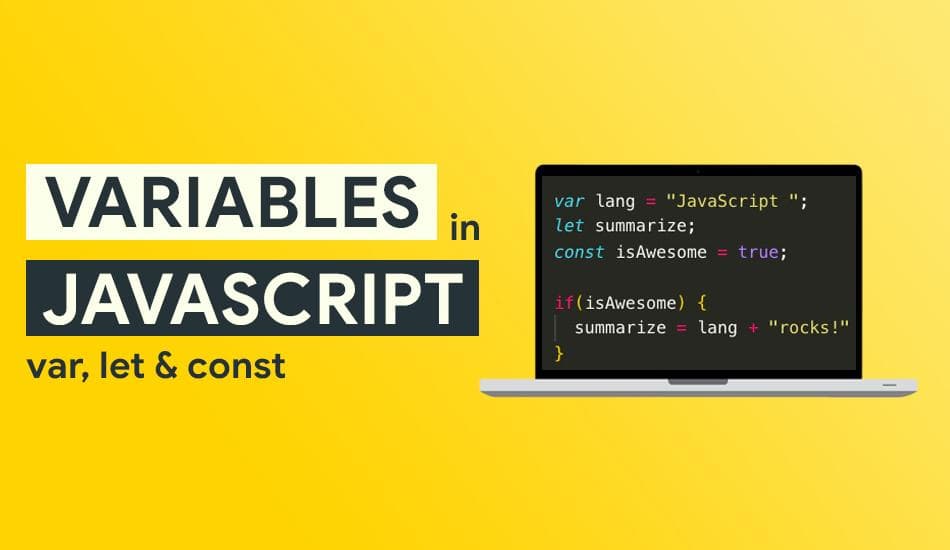
Javascript has evolved a lot over the last years before it was only one way to declare a variable. Now there are multiple ways, in this article, we will go over var, let, const and explain the difference and when you should use them.
Var
var car = "BMW";
console.log(car); // BMW
Here we first declare a variable named car and set it to be the string "BMW" and finally print it out, nothing strange here. This was the standard way to declare a variable for many years.
Let's talk about three important things to be aware of.
The initial value of a variable declared with var is Undefined
var person;
console.log(person); // undefined
If we don't set a value for the variable it will be set to Undefined.
variables declared with var are hoisted
console.log(house); // undefined
var house = "treeHouse";
wait what's going on here? Yes, this is one of the weird quirks with Javascript. Under the hood, JavaScript moves up all var declarations to the top of the current scope. So instead of throwing an error, it will return undefined when you try to access the variable.
Variables declared with var will have function scope not block scope
function printFact() {
var topic = "JavaScript";
console.log("Did you know " + topic + " is awesome?");
}
printFact(); // Did you know JavaScript is awesome?
console.log(topic); // Uncaught ReferenceError: topic is not defined
If you try to access topic outside the printFact variable, it will throw an error. Uncaught ReferenceError: topic is not defined
What about if statements?
if(true) {
var name = "Patrik";
}
console.log(name); // Patrik
As you can see we can accesss name outside the if statement. var has function scope not block scope.
let and const
With es2015 (es6) two new ways of declaring variables were introduced. so with three different ways to declare a variable, you might be asking which one should I use then? Let's start with let and then look at how const differ.
let
let have block scope that, that means it won't be accessible outside the block.
function printCar() {
let car ="Volvo";
console.log(car); // Volvo
}
if(true) {
let car = "Ford";
console.log(car); // Ford
}
console.log(car); // throw error
Three things to note here, car variable insidePrint car cannot be assessable outside the function.
Second car variable inside the if statement is not the same variable as in printCar, it lives in its own scope.
the car variable inside the if statement cannot be accessible outside the brackets. Remember with var? it was then possible.
const
Okay so hopefully you got the hang of var and let now, but what about const? const always reference the same object or primitive value, so what does that mean? it means we can not reassign it a new value.
const bestFriend = "Tom";
bestFriend = "Robin"; // TypeError: Assignment to constant variable.
const about = {
age: 28,
profession: "programmer";
}
about.age = 39;
about.canFly = false;
about = {
favoriteFood: "Spagetti"
}; // TypeError: Assignment to constant variable.
First, we declare bestFriend with the value "Tom", after that we try to change it to "Robin" that will throw an error, the reason for that is we are reassigning it a new value.
Let's take a look at how an object works, we can edit existing properties and add new properties without any problem. But when we try assign a new object to the about variable it will throw an error.
Arrays work the same, we can change the value inside the array, but we can not reassign it a new array, or any other types for that matter.
Which one should I use?
Should I use var?
As of 2019, it's safe to say you should not use var as long as you are not developing for very old browsers / old environments or you have a very valid reason for it (do they actually exist?). You might still see it in code out there, so it's important to know how it works.
Should I use let or const?
It depends, but if you know your variable will not be assigned a new value, use const else use let. It's considering a best practice to stick with const as much as possible to reduce bugs.